Generally, you code with a high-level language. A high-level language is one that we humans can understand more easily.
The computer does not, however, understand high-level languages. It only understands programs written in 0's and 1's in binary - this is called machine code.
To convert source code into machine code, you use either a compiler, an interpreter or a mix of both (Jiter Languages).
There are differences between all of these three approaches. Neither approach has a definite advantage over the other - if one approach was always better, it is likely that we would have already begun to use it everywhere, and we would have way less programming languages.
Compiler Languages
How does a Compiler work?
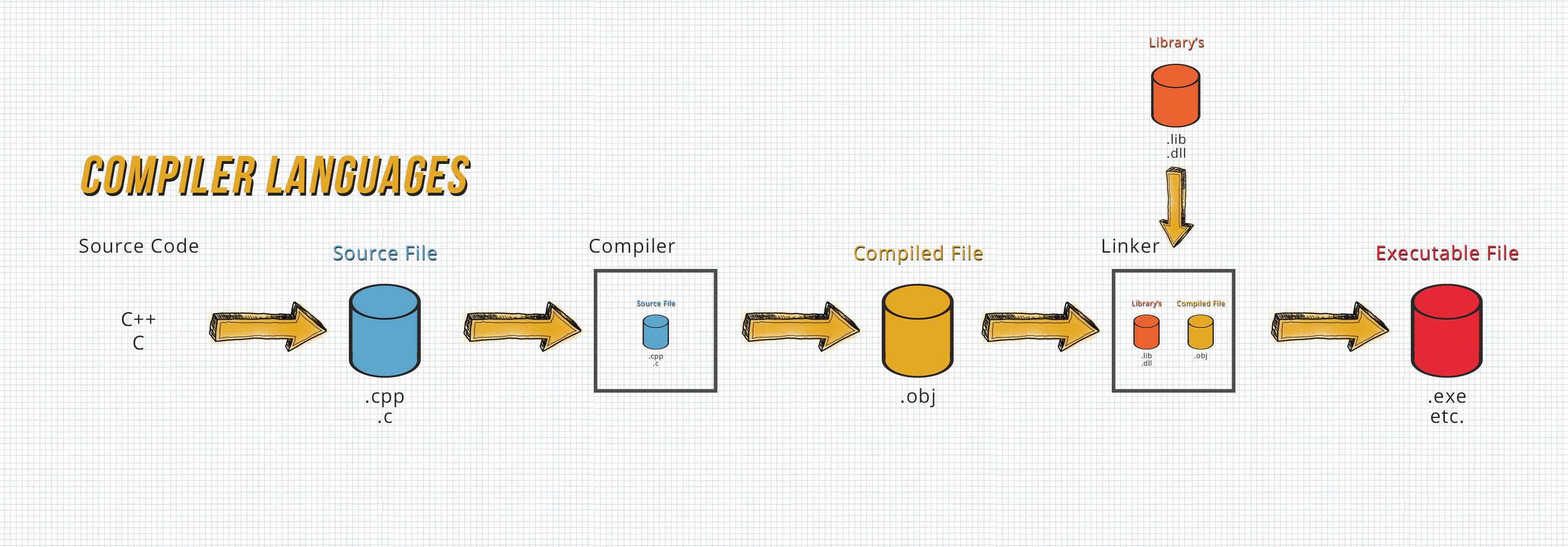
Above is a good graphic that describes more visually, how most compilers function. But now let's get to the less visual way đ.
- The Source File / Source Code - a .CPP or .C file for example, gets put into the Compiler.
- This Compiler then verifies syntax and semantics of the whole source file according to the language and then transforms the whole input file input into Assembly Code and then uses an Assembler to convert the Assembly code to machine language which is stored in Object Files (.obj). By the way, this Machine Language differs from CPU to CPU because they are all physically different. Every CPU has a different Assembler which is provided by the CPU Manufacturer.
- After that, the Linker takes the Object File and adds/links additional Librarys (.lib), .DLL or similar which contain functions that you may have used and called in your program. At this step the Linker also checks if you may have called Library functions that donât even exist.
- Now the linker outputs the executable that you are used to (.exe) and that the CPU can run.
But watch out! The word âcompilerâ does not automatically mean that it outputs a lower level machine code
Generally speaking, a compiler is just a program that translates one programming language (Source Language) into an equivalent program in another language (target Language).
There are also so-called Source-to-Source Compilers that convert one High Level Programming Language into another High Level Programming Language - you can find a Python to C# Compiler here for example.
Interpreter Languages
How does an Interpreter work?
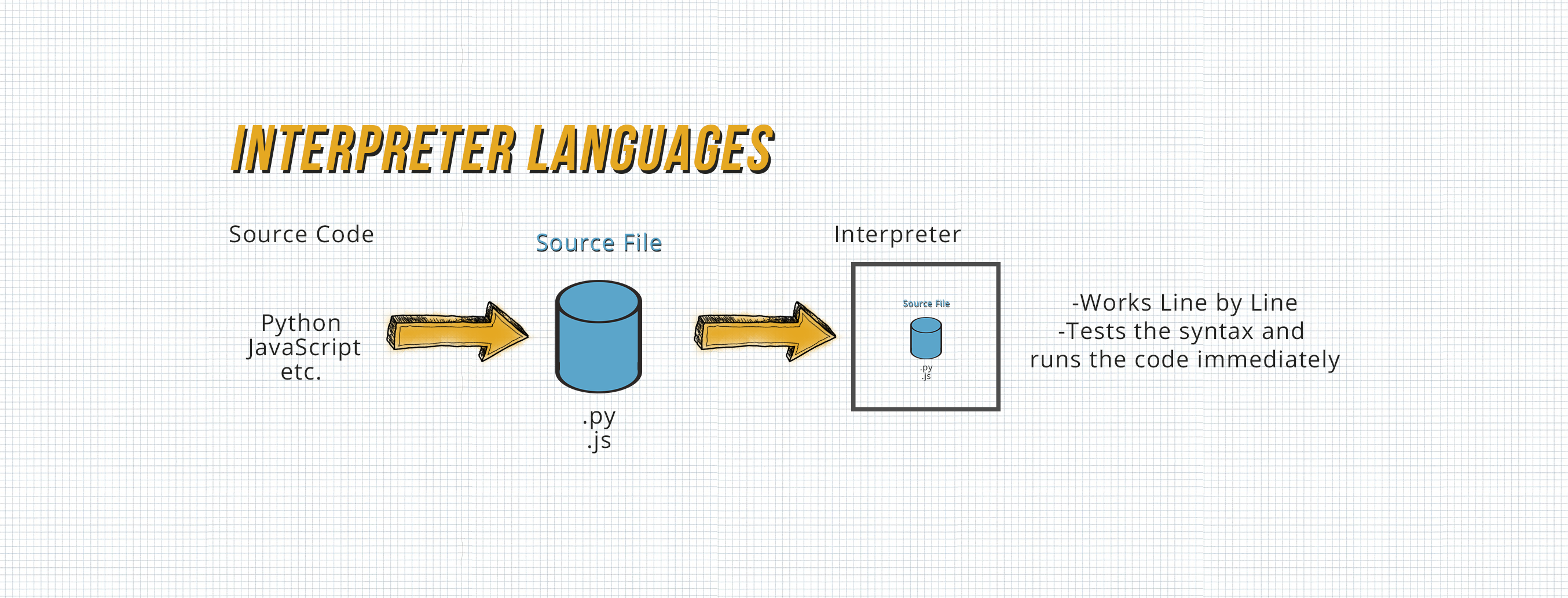
An Interpreter also translates Source Language into an equivalent program in another target Language.
But the Key difference to a Compiler is that it goes through Source Code line by line, verifies the lines syntax and semantics according to the language and then runs this line instantly before going to the next line and doing it again.
By the way, this running line by line thing instead of checking everything beforehand is also why a Compiler will give you almost all errors at once before running, but an interpreter will run your code till it hits the instruction you have written wrong.
But I am drifting away, I will get into the Pros and Cons more thoroughly down below by comparing each language type to each other.
Jiter Languages
How do Jiter Language work?
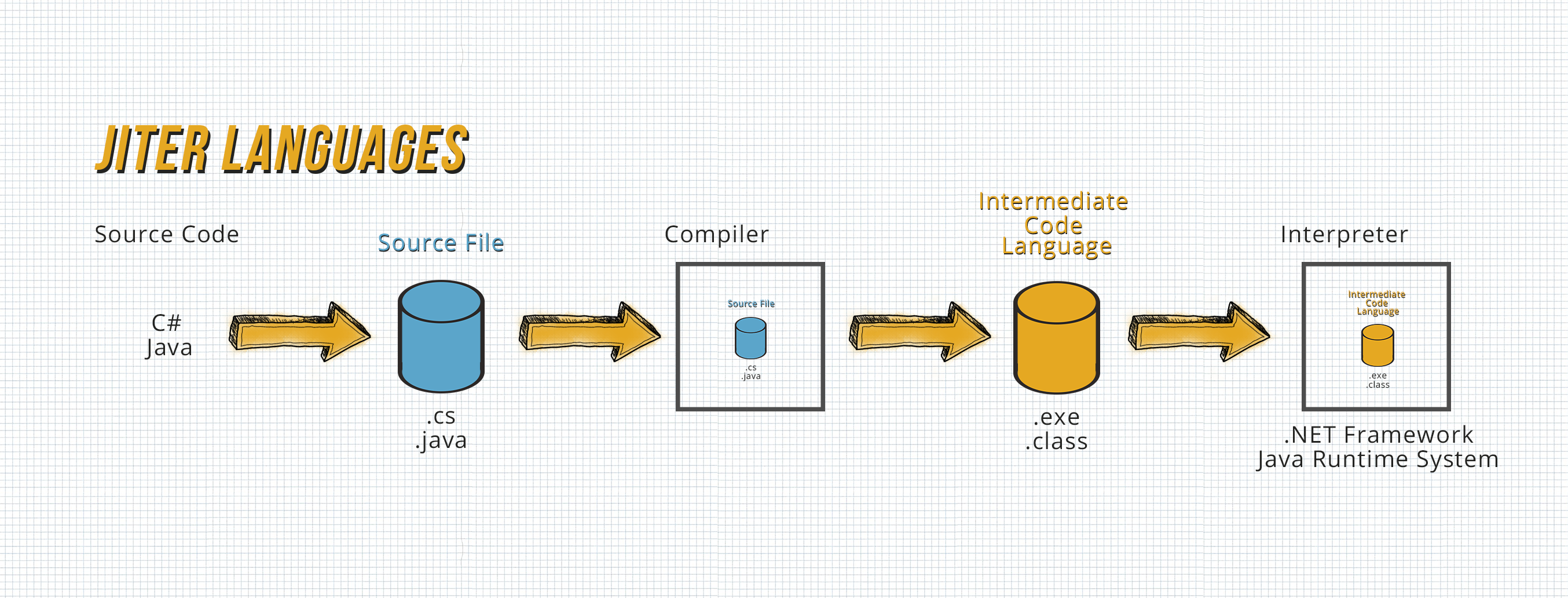
This third programming language type is a mixture of concepts used in a Compiler and an Interpreter.
A Jiter Language works likes this:
- The Source File / Source Code - a .CS or .java file for example, gets put into the Compiler.
- This Compiler then verifies syntax and semantics of the whole source file according to the language and then transforms the whole input file input into an Intermediate Code Language - often referred to as âIL Codeâ. This Intermediate Code is CPU independent, but can also not be run by the CPU. The next step is needed for that.
- Now at runtime (when the user executes your Program) an Interpreter - .NET Framework or the Java Runtime System that has to be installed on the user's Computer takes the Intermediate Code, translates it to native code which your CPU can understand and runs it.
This concept of an Intermediate Code Language enables you to write in many High level languages with the same interpreter being able to run it.
The .NET Framework for example, allows you to write in C#, F#, Visual Basic .NET and many others, but you only need the ONE .NET Framework to run them all.
Comparison Between Programming Language Types
To summarize again, the difference between Compiler, Interpreter and Jiter programming languages is actually only when and how the source code is converted into Machine Code.
In the case of compiler languages, the code is compiled once by the developer.
In interpreter languages, the code is "compiled" (converted into machine code) by the end user each time it is executed.
And with Jiter languages, the developer converts the source code into an intermediate code language, which is then later converted into machine code by the end user at runtime.
Of course, all three methods have their own advantages and disadvantages, which are listed in the following table:
Advantages | Disadvantages | |
Compiler Languages | -Faster (At Runtime) -No need for additional Software to run executable -Harder to reverse engineer | -Slower (While Compiling) -OS dependent -High Level Code can't be changed without having to recompile (You can of course Change the Assembly Code without having to recompile, check out this article) |
Interpreter Languages | -Faster (While Compiling [There is no Compiling]) -OS Independent -Syntax errors are only noticed during the runtime (This is a Pro because this makes it easier to debug and find the exact position of a error, but it can also be seen as a Con) -Code can be changed during runtime | -Syntax errors are only noticed during the runtime -More âopen to the worldâ which makes reverse engineering easier |
Jiter Languages | -OS Independent (As long as the Interpreter exists for your OS) | -Intermediate Code takes up a lot of memory -More âopen to the worldâ which makes reverse engineering easier |
Not Programming Languages
Why is HTML not a programming language?
Simple Answer:
HTML does nothing, remembers nothing, computes nothing, decides nothing, writes nothing and reads nothing. It is a markup language, a way to store like data and tell info about that data.
HTML encapsulates, or âmarks upâ data within HTML tags, which define the data and describe its purpose on the webpage. The web browser then reads the HTML, which tells it things like which parts are headings, which parts are paragraphs, which parts are links, etc. The HTML describes the data to the browser, and the browser then displays the data accordingly.
On the other hand programming languages are used to do something, store variables, decides things, write and read data.
Now the more technical explanation:
HTML is missing the three (maybe only two - depends on how you see it) Control structures that make a programming language a programming language. The three Control structures are Sequence, Selection and Iteration
Sequence:
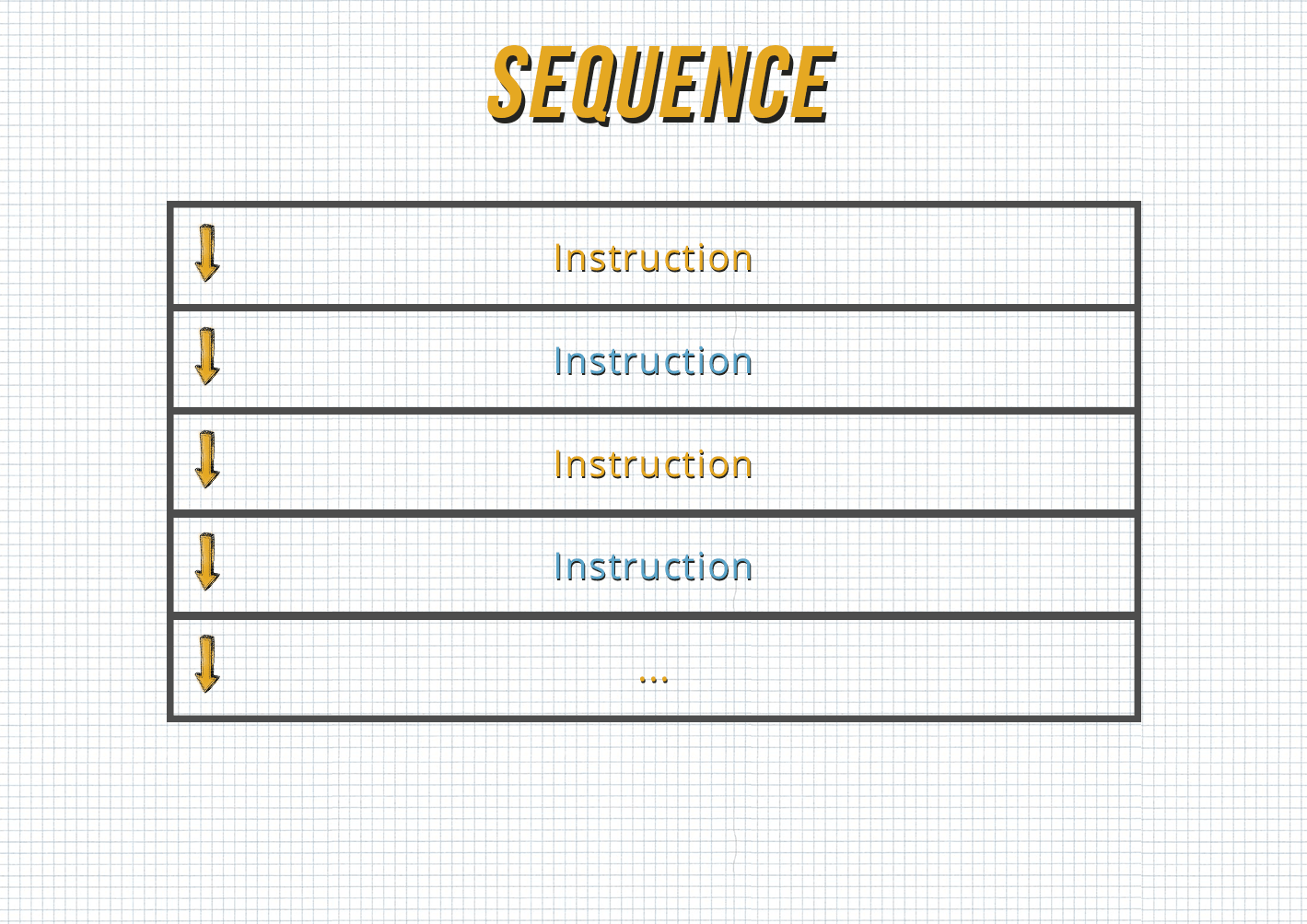
Sequential execution is when statements are executed in a given order, it follows a serial or sequential flow in which the flow depends on the series of instructions given to the computer.
Unless a special sequence is defined, the Instructions get executed in the obvious sequence - one after another.
This control structure is what I meant when I said that HTML may just be lacking two of the three control structures. That's because you might argue that, because HTML is read from top to bottom, that it has a sequence.
Selection:
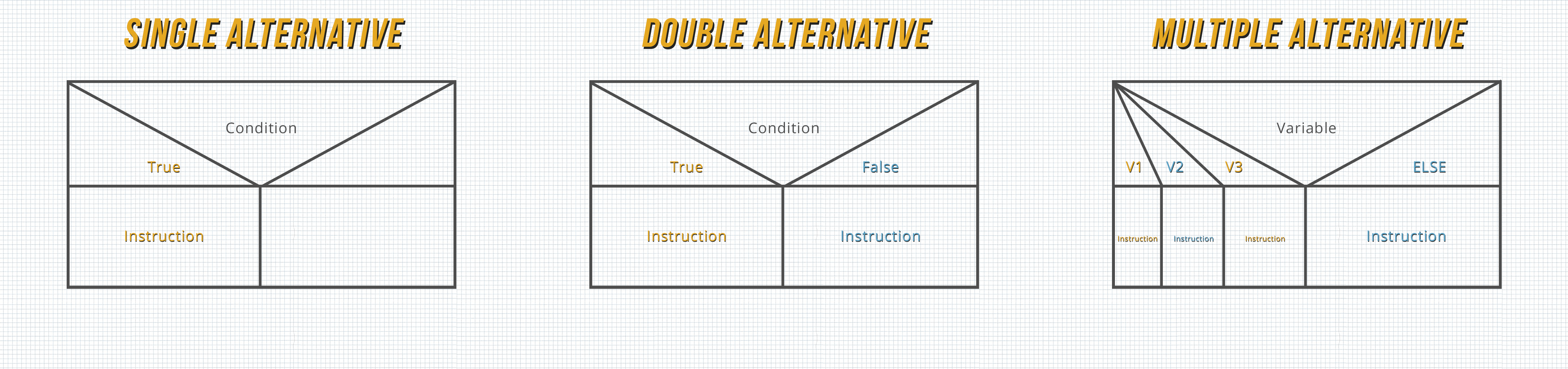
Selection is the process of the Computer deciding which code to execute and which code not to execute based on some conditions that have to be met.
The structures that use this type of logic are known as Conditional Structures. There are three types of Conditional Structures - They can be seen in the above image as a Chart and implemented in the following ways:
Single Alternative:
If (Condition) then: [Instruction A] EndIF
Double Alternative:
If (Condition), then: [Instruction A] Else: [Instruction B] EndIF
Multiple Alternatives:
switch (variable) case (Condition Value): [Instruction A] break case (Condition Value): [Instruction B] break else: [Instruction C] break;
There is definitely no "selection" control structure in HTML as there are no if statements or similar, which means that HTML is missing the crucial âselectionâ control structure that is needed for a Programming Language to count as a Programming Language.
Iteration:
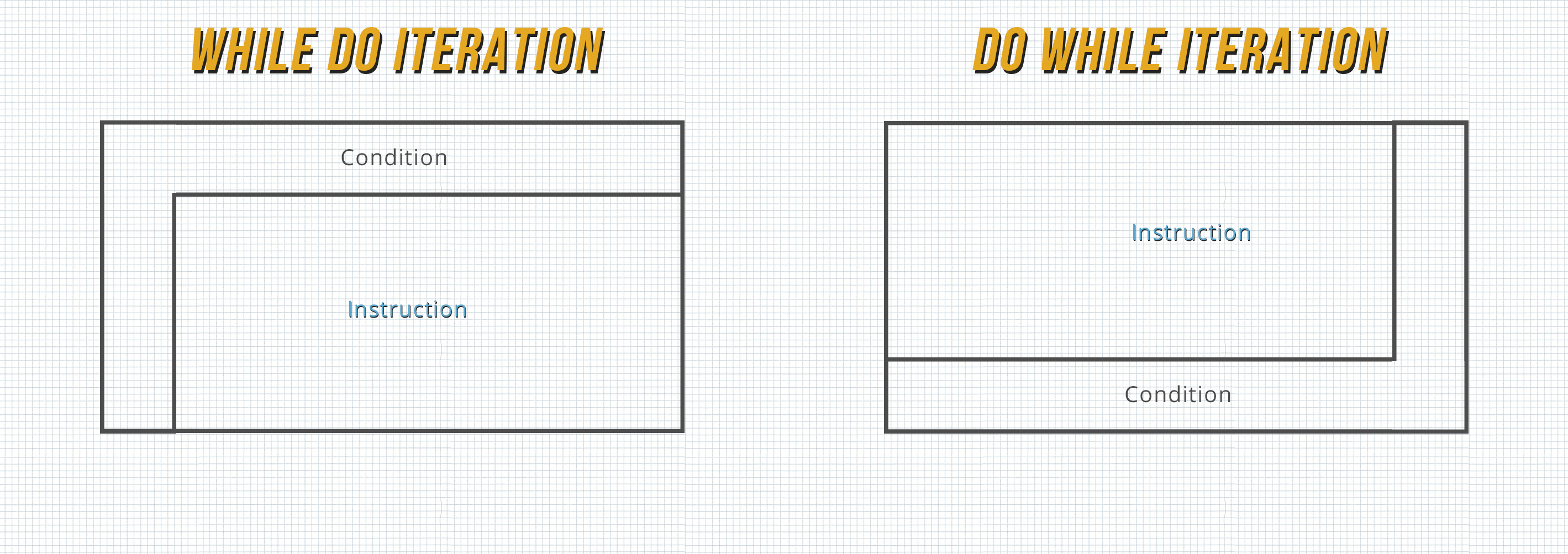
Iteration is nothing more than the automatic process of repeating the execution of certain code a certain number of times
Iteration (or looping) gives computers much of their power. They can repeat a sequence of steps as often as necessary without you having to write the code multiple times.
There are two types of Iteration, the âWhile Do structureâ that first checks if the condition is met and then only executes code and the âDo While structureâ that executes code at least once and then checks before executing the second time if the condition is met - (By the way, for loops count as âWhile Do Structuresâ).
See the following code examples to see how these two types of loops can be implemented:
While Do Structure:
Repeat while Condition: [Instruction] EndLoop
Do While Structure:
[Instruction] Repeat while Condition EndLoop
There are certainly no loops or similar present in HTML, which means that HTML is also missing the critical âIterationâ Control structure that makes a Programming Language a Programming Language.